Overview
FlashCards is a desktop flashcard consolidation application for students to compile and organise notes for different subjects. It provides an easy way to have a simple way to store different notes The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: added Toggle feature
-
What it does: It allows the user to navigate between flashcard easily with a next and previous command.
-
Justification: This feature improves the navigation process for viewing the flashcard. Instead of always selecting a flashcard by index, users can just command next or previous to toggle through the flashbook.
-
Highlights: This enhancement is designed to work with existing commands as well as other commands that will be added in the future. It incorporates algorithms and operating styles thought in this module.
-
-
Major enhancement: added Sort feature
-
What it does: It allows the user to view all flashcards based off their desired difficulty.
-
Justification: This feature provides a filtered flashbook for users to focus on only reading flashcards with selected difficulty. This commands also allow users to search for flashcards of that difficulty.
-
Highlights: This enhancement is designed to work with existing commands as well as other commands that will be added in the future. It focuses mainly on the logic and model segment of the code to produce the desired outcome. I had to use various Java built-in libraries to achieve this.
-
Credits: This code was inspired by the find command that was provided in address book 4. I adapted from
TopicContainsKeywordPredicate
class, made changes to it to achieve the desired outcome.
-
-
Minor enhancement: Added a status bar for total number of flashcards that the flashbook contains.
-
Minor enhancement: Added an Events Center for tracking any addition to the code.
-
Minor enhancement: Added a
selectSubject
command to allow users to choose the subject. -
Code contributed: [Functional code]
-
Other contributions:
-
Project management:
-
Enhancements to existing features:
-
Testing:
-
Documentation:
-
Community:
-
{you can add/remove categories in the list above}
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Viewing the next flashcard: next
or n
Views the next flashcard in line.
Format: next
or n
-
Selects the next flashcard and displays its content
Steps to view next flashcard:
-
First select the subject of the flashcards you want to toggle with
selectSubject
command. -
Next, use the
select
command (see Section 3.9) to select a flashcard first. -
Use
next
/n
command to go to the next flashcard.
Examples:
-
selectSubject math
Selects all flashcards that is tagged under subject math.
select 2
Selects the 2nd flashcard in the filtered list.
next
Selects the next flashcard in the list (i.e. the 3rd flashcard); and displays the content of this flashcard.+ -
selectSubject science
Selects all flashcards that is tagged under subject science.
select 5
Selects the 5th flashcard in the list.
n
Displays the next flashcard in the list (i.e. the 6th flashcard); and + displays the content of this flashcard.
The next flashcard in line will be selected and its content will be displayed.
Viewing the next flashcard: previous
or p
Views the previous flashcard in line.
Format: previous
or p
-
Selects the previous flashcard and displays its content
Steps to view previous flashcard:
-
First select the subject of the flashcards you want to toggle with
selectSubject
command. -
Use the
select
command (see Section 3.9) to select a flashcard first. -
Use this
previous
/p
command to go to the previous flashcard.
Examples:
-
selectSubject math
Selects all flashcards that is tagged under subject math.
select 2
Selects the 2nd flashcard in the list.
previous
Selects the previous flashcard in the filtered list (i.e. the 1st flashcard); and displays the content of this flashcard. -
selectSubject science
Selects all flashcards that is tagged under subject science.
select 5
Selects the 5th flashcard in the list.
p
Selects the previous flashcard in the list (i.e. the 4th flashcard); and displays the content of this flashcard.
The previous flashcard in line will be selected and its content will be displayed.
Selecting a flashcard: sort DIFFICULTY
Displays list of flashcards under the chosen difficulty.
Format: sort DIFFICULTY
-
DIFFICULTY
: Difficulty level of the flashcard to be selected. This difficulty will be shown in the details of each flashcard. Must be a positive integer such as 1, 2, 3 only.
Steps to select a flashcard:
-
Click on a subject in the subjects panel; or
-
Enter the
selectSubject
command to select a subject (see Section 3.9.); then -
Use the
sort
command to filter the list of flashcards with selected difficulty level
Examples:
-
After clicking on a subject e.g. Math, flashcards that are tagged under "Math" will be displayed in the flashcards panel.
Next, enterselect 2
to select the 2nd flashcard of the subject "Math" in the flashcards panel. -
After entering this command
selectSubject English
, all flashcards that are tagged under "English" will be displayed in the flashcards panel.
Next, enterselect 1
to select the 1st flashcard of the subject "English" in the flashcards panel.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Toggle feature
Current Implementation
The toggling feature is mainly facilitated by 2 classes, NextCommand
and PreviousCommand
. For either commands to work, SelectCommand
is first executed to retrieve the choice of Index
of the flashcard to begin toggling.
Following that, NextCommand
or PreviousCommand
can be called interchangeably to toggle the flashcard being displayed in the rightmost panel.
For NextCommand
, the following operations are implemented:
-
NextCommand#getNextIndex(Index index)
— retrieves the current flashcard’s Index fromSelectCommand
class. -
NextCommand#setNextIndex(Index index, int start)
— pass the index currently being used inNextCommand
toPreviousCommand
.
Similarly, for PreviousCommand
, the following operations are implemented:
-
PreviousCommand#getPreviousIndex(Index index)
— retrieves the current flashcard’s Index fromSelectCommand
class. -
PreviousCommand#setPreviousIndex(Index index, int start)
— pass the index currently being used inPreviousCommand
toNextCommand
.
The sequence diagram below illustrates the execution of SelectCommand
and how the index will be passed to both NextCommand
and PreviousCommand
class.
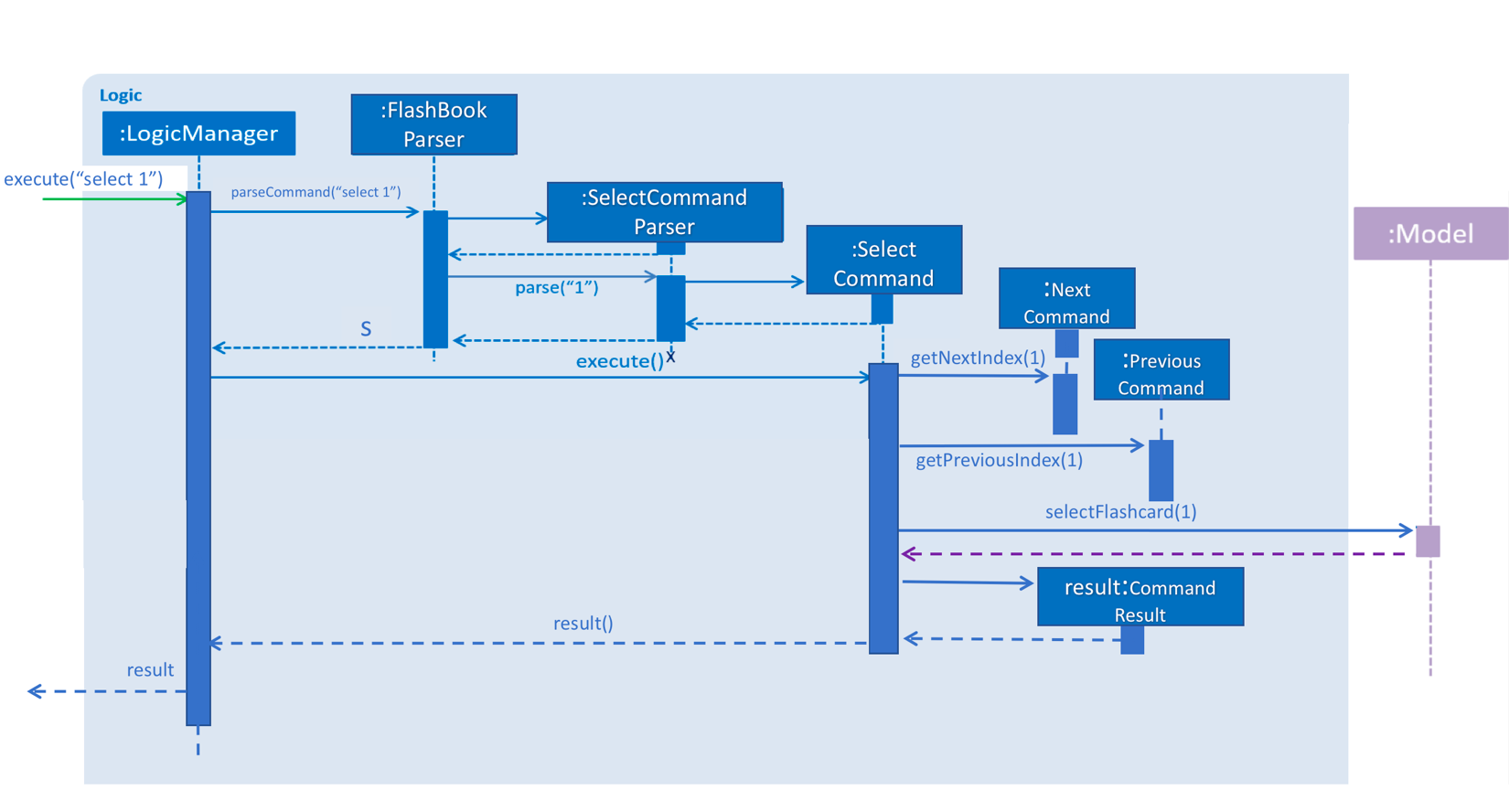
After SelectCommand
is being called, NextCommand
or PreviousCommand
can be called interchangeably.
The sequence diagram belows illustrates the execution of NextCommand
and how the index value will be passed to PreviousCommand
class.
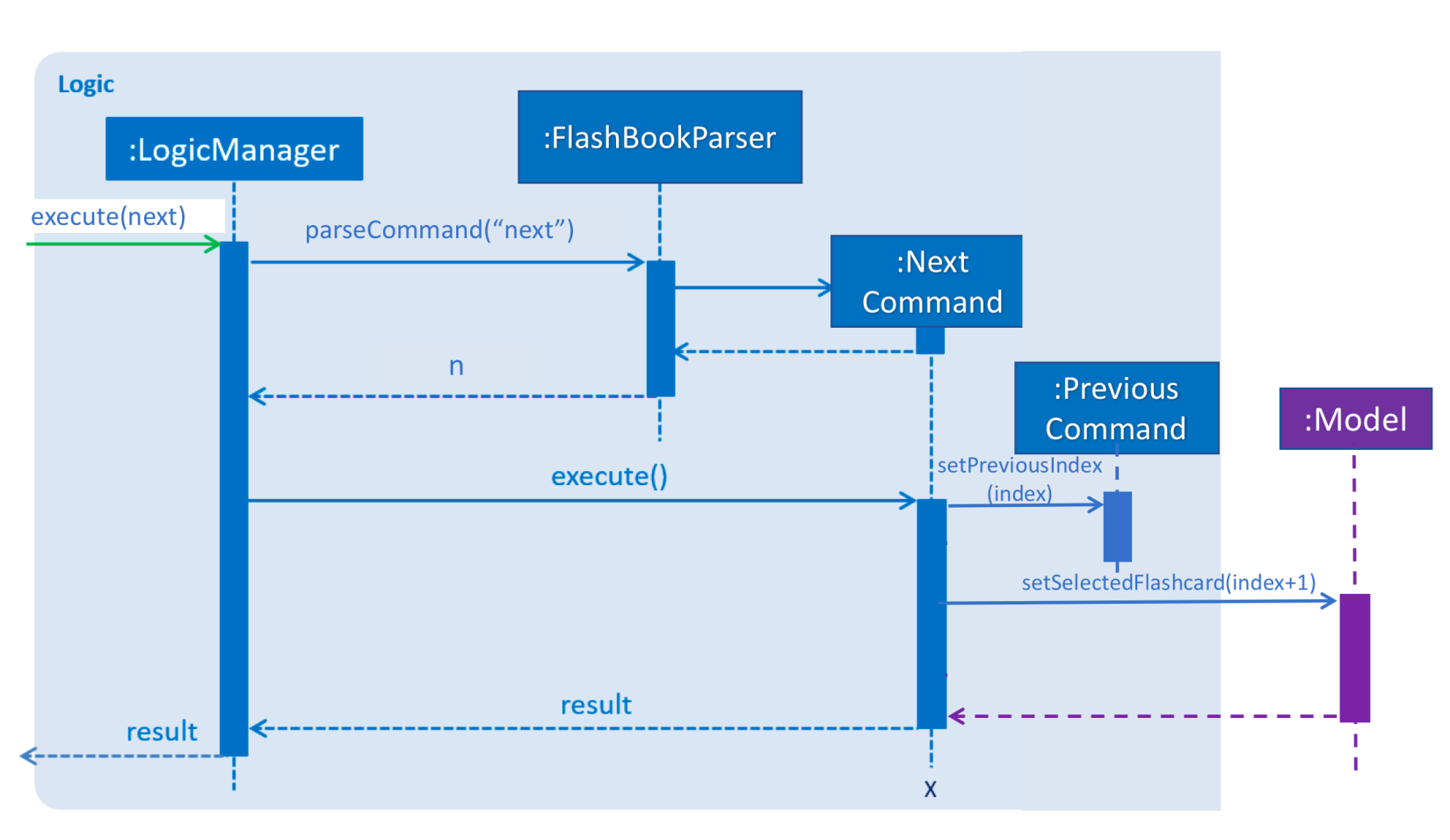
To execute toggling of next flashcard, execute the following commands:
1) select 2
[2 is just an example, choose index of choice]
2) next
Flashcard with next 3 will be displayed for the example above.
The sequence diagram below illustrates the execution of PreviousCommand
and how the index value will be passed to NextCommand
class.
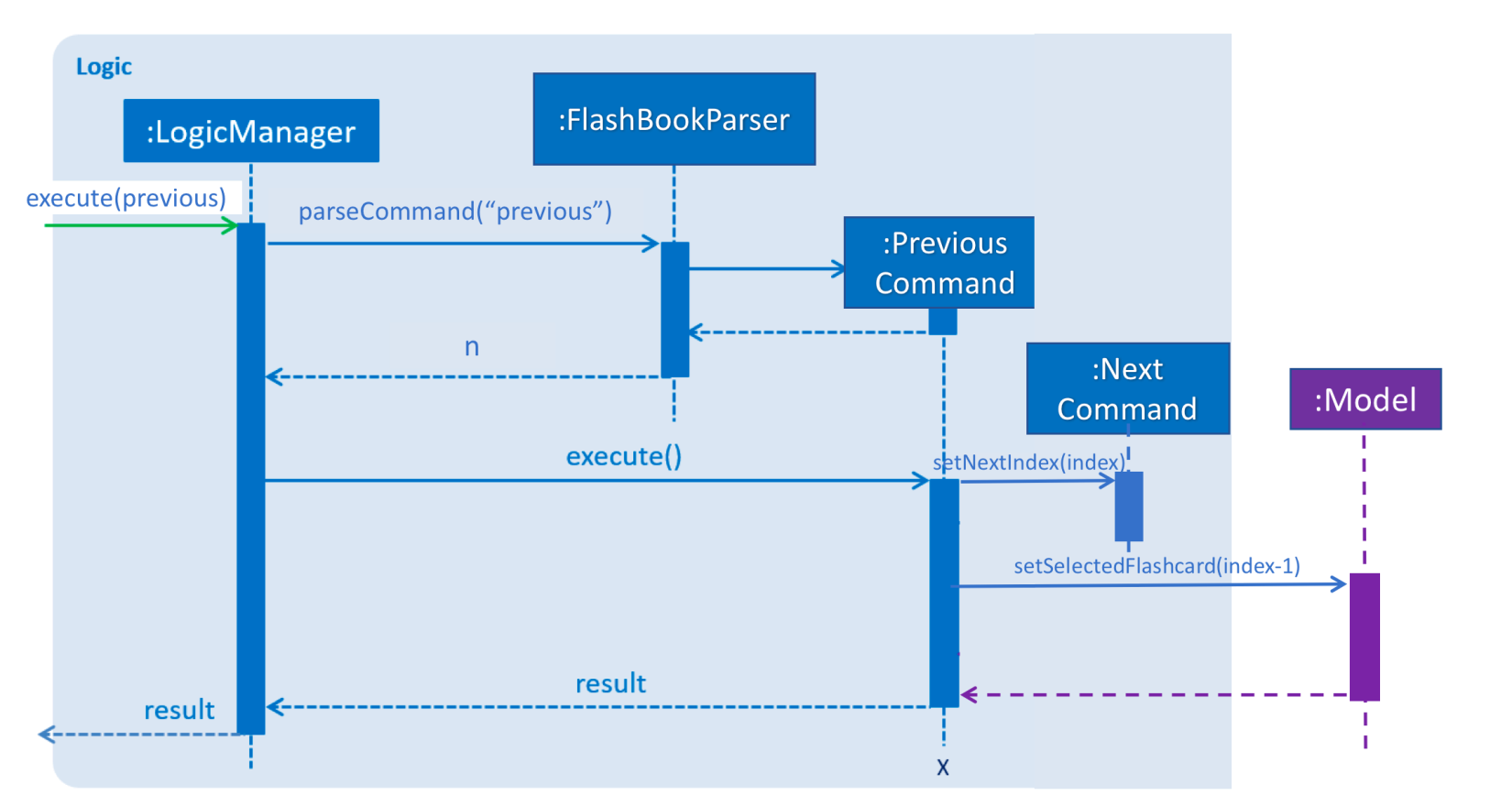
To execute toggling of previous flashcard, execute the following commands:
1) select 2
[2 is just an example, choose index of choice]
2) previous
Flashcard with next 1 will be displayed for the example above.
An example scenario of selecting the flashcard (Step 1) and executing the next or previous after select (Step 2) or otherwise (Step 3) is shown below.
Step 1: Selecting a Flashcard
-
Executing a
select INDEX
command. -
The
INDEX
will be passed into Next Command, facilitated bygetNextIndex()
in NextCommand class. In NextCommand class,nextIndex
will be initiated withINDEX+1
to obtain the index number of the next flashcard. -
The
INDEX
will also be passed into Previous Command, facilitated bygetPreviousIndex()
in PreviousCommand class. In PreviousCommand class, thepreviousIndex
will be initiated withINDEX-1
to obtain the index number of the previous flashcard.
Step 2: Executing next or previous after select
The following is an execution of NextCommand:
-
As mentioned in step 1,
nextIndex
has been initiated with the index obtain through Select command. -
When NextCommand is called, the
nextIndex
will be passed into Previous Command, facilitated bysetPreviousIndex()
in PreviousCommand class. In PreviousCommand class, thepreviousIndex
will now be replaced bynextIndex -1
to obtain the index number of the previous flashcard. -
Lastly nextCommand will set the flashcard of
nextIndex
, facilitated by setSelectedFlashcard() in model. -
Thereafter, next or previous can be called interchangeably without having to select a flashcard.
The following is an execution of PreviousCommand:
-
As mentioned in step 1,
previousIndex
has been initiated with the index obtain through Select command. -
When PreviousCommand is called, the
previousIndex
will be passed into Next Command, facilitated bysetNextIndex()
in NextCommand class. In NextCommand class, thenextIndex
will now be replaced bypreviousIndex+1
to obtain the index number of the next flashcard. -
Lastly PreviousCommand will set flashcard of
previousIndex
, facilitated by setSelectedFlashcard() in model. -
Thereafter, next or previous can be called interchangeably without having to select a flashcard.
Design Considerations
-
Alternative 1 (current choice): Obtain the index after select command.
-
Pros: Easy to implement and convenient for the user.
-
Cons: Have to manually select the first card to choose from.
-
-
Alternative 2: Toggling will begin from the first index.
-
Pros: Saves the user the step of having to select the index of choice.
-
Cons: Might be too troublesome for some users who wants to being from a flashcard index that is large.
-
Quizzing by Difficulty
This mode will be largely focused on viewing flashcard based on a chosen difficulty. Flashcards are added with a difficulty level set by the user. The difficulty level can be toggled in this mode for the user to focus more on difficult concepts or easier concepts. This mode can be used along side "Subject" Testing Mode
to get a further filtered list of flashcards with both chosen subject and difficulty.
Current Implementation
This mode of quizzing is facilitated by SortCommand
. This command filters all the flashcard with the same difficulty level.
When a flashcard is added, user must input a difficulty level 1 (easy), 2 (medium) or 3 (difficult) for each flashcard.
The SortCommand
will help students to focus on one chosen difficulty level, helping them prioritize their time well.
The sequence diagram below illustrate how SortCommand
is executed.
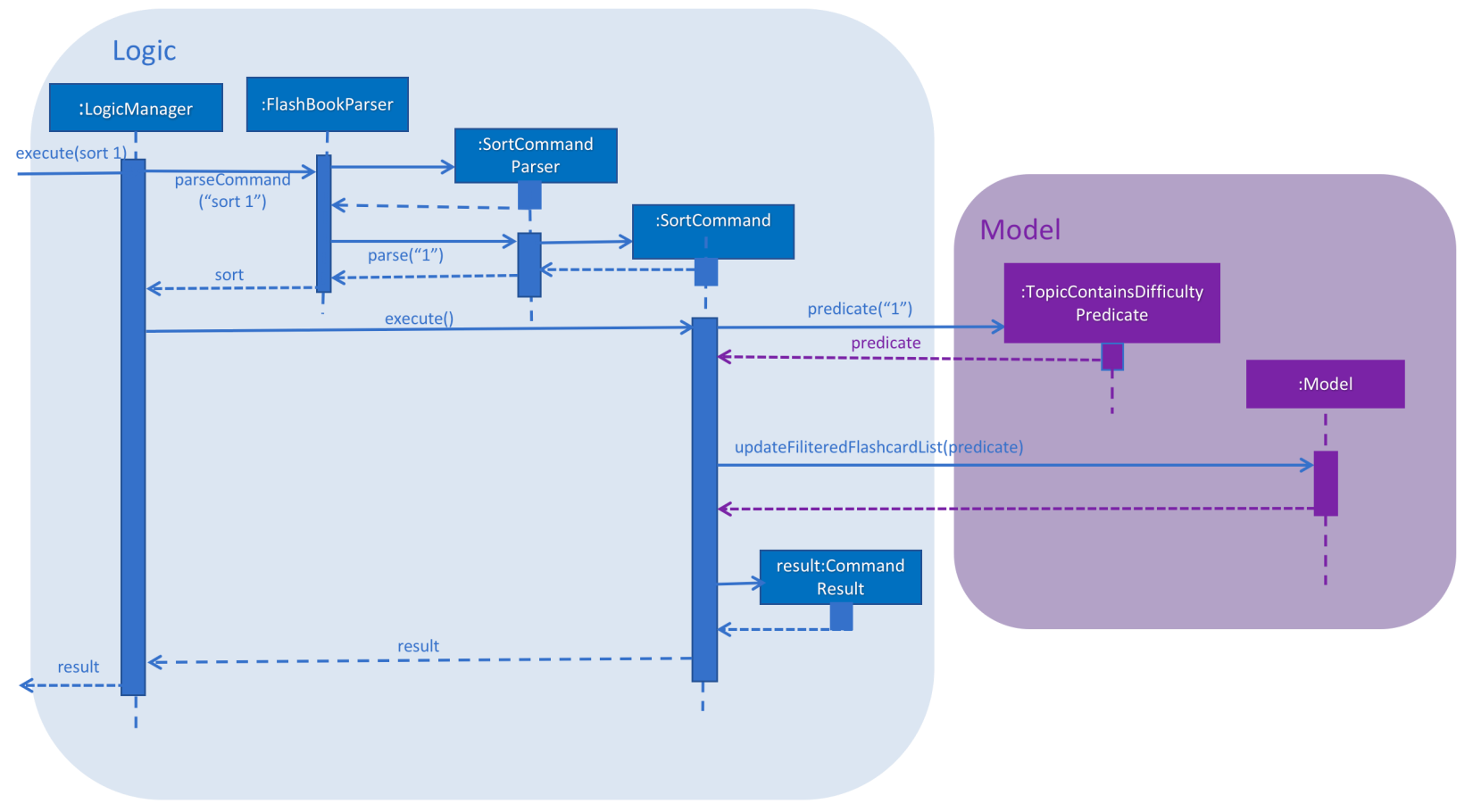
Design Considerations
-
Alternative 1 (current choice): Filters flashcard and displays all flashcard with chosen difficulty.
-
Pros: Easy to implement.
-
Cons: Only get to see flashcards under 1 difficulty level.
-
-
Alternative 2: Displays flashcards in ascending or descending difficulty level.
-
Pros: Can view more flashcards at once.
-
Cons: Difficult to implement.
-