Overview
This portfolio is written to document the process of developing FlashCards throughout the course of one semester in a team of 3 members. My team chose to morph the basic Address Book into FlashCards and my role was to design and write the code for the deadline features as well as the GUI display of the flashcards.
FlashCards is a desktop flash book application written in Java which targets mainly secondary school students. It is specially designed to cater to users a platform to digitize and store their notes in one place. The user interacts with the application using a Command Line Interface (CLI) and it has a Graphical User Interface (GUI) created with JavaFX.
The following sections illustrate these enhancements in more details, as well as the relevant sections I have added to the user and developer guides in relation to these enhancements.
Summary of contributions
This sections summarises my coding, documentation and other relevant contributions to the team project.
-
Major enhancement 1: added the ability to add deadlines to flashcards (Pull requests #12, #13, #14)
-
What it does: The deadline command allows the user to add deadline or exam dates to a specified flashcard. The feature also includes an internal Calendar which will count the number of days left before the deadline and display it below the deadline.
-
Justification: This feature improves the product significantly as it serves as a reminder to the user about the upcoming deadlines or exams.
-
Highlights: This enhancement does not affect existing commands and commands to be added in future. It is an independent command and it can only be added after the selected flashcards exist in the FlashBook. This gives the user the choice to decide if he/she wants to assign a deadline to the flashcard. Also, if the user wants to update the existing deadline, he can enter the command again with a different date and this new date will override the existing date. Implementation of the command was simple and straightforward, however, implementing the Java.util.Calendar together with it was a challenge as the system has to check if the date entered is valid before accepting it.
-
Credits: Help from [Oracle Java Class Calendar]
-
-
Minor enhancement: Added tests for deadline command.
-
Code contributed: [Functional code] [Test code]
-
Major enhancement 2: added GUI display of topic and content (Pull request #80)
-
What it does: The GUI display allows the user to alternate between the topic and the content of the flashcard.
-
Justification: The physical copy of a flashcard has two sides, and to simulate that in the application, I added two buttons for topic and content respectively. This allows the user to switch between topic and content easily.
-
Highlights: -
-
Credits: -
-
-
Minor enhancement: Changed the background of GUI display to black for the text to stand out (Pull request #86)
-
Code contributed: [Functional code in Java] [Functional code in FXML]
-
Other contributions:
-
Project management:
-
Managed releases version 1.2 to 1.3 (2 releases) on Github.
-
Set up relevant project repositories (e.g. Organisation repository, Issue Tracker, Travis, Coveralls)
-
-
Enhancements to existing features:
-
Added alias and relevant tests for 5 commands (Pull requests #9)
-
Added new StackPane and split the window into 3 placeholders (Pull request #30)
-
Refactored Subjects to Flashcards (Pull requests #43)
-
Refactored Name to Topic, Phone to Difficulty, Address to Content and AddressBook to FlashBook (Pull requests #45
-
Edited relevant fields (Email) (Pull request #46)
-
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide: (Pull requests #3, #9, #10, #20, #45)
-
Did cosmetic tweaks to existing contents of the Developer Guide: (Pull requests #3, #8, #43, #45, #86)
-
Added relevant information and links to relevant documentations (e.g. Build.gradle, README etc) (Pull request #3, #4, #16, #18)
-
Managed relevant documentations (e.g. User Guide, Developer Guide etc.) by ensuring everything is well-documented.
-
-
Community:
-
Tools: -
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Adding a Deadline/Note to a flashcard: deadline
or note
Adds a deadline or notes to the flashcard.
Format: deadline INDEX d/DEADLINE
or note INDEX d/NOTE
-
DEADLINE
: Deadline of flashcard. -
NOTE
: Important notes to be added.
Examples:
-
deadline 1 d/12 January 2020
Adds a deadline "12 January 2020" into the flashcard with index 1. -
note 3 d/Clarify with Teacher
Adds a note into the flashcard with content "Clarify with Teacher".
In the case that a new date is to be added to a flashcard with an existing deadline or note, corrections can be made by overwriting the existing information with the same commands.
Deleting a subject: deleteSubject
Deletes the specified subject.
Format: deleteSubject SUBJECT
-
SUBJECT
: Subject to be deleted. This subject name can be found in the displayed list of subjects on the left panel. Must be a subject that is already in the subject list
Examples:
-
deleteSubject Chemistry
All flashcards that are tagged under "Chemistry" will be deleted.
Note This action is not reversible. The undo
and redo
command do not apply to the deletion of subjects.
Clearing all flashcards: clear
or c
Clears all flashcards.
Format: clear
or c
Examples:
-
clear
All flashcards that are in the FlashBook will be deleted.
Note This action is not reversible. The undo
and redo
command do not apply to the clearing of flashcards and subjects simultaneously.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
-
Came up with the three features which were further implemented by my team mates.
Shelf-Life of FlashCards
Current Implementation
The user can determine set a deadline for the flashcards and determine how long the flash cards will exist in the database “FlashCards”. The following operations are available:
-
FlashCards#deadline()
orFlashCards#note()
— Adds a deadline/note for the specified flashcard. -
FlashCards#deleteSubject()
— Deletes a specific subject when in "Subject" Knowledge Testing Mode. -
FlashCards#clear()
— Clears the entire database.
These operations are exposed in the Logic
interface as Logic#deadline()
, Logic#note()
, Logic#deleteSubject()
and Logic#clear()
respectively.
Deadline Command
For the deadline
command, the user can input a date whereby the flashcards would be unavailable thereafter.
Step 1. The user can input a deadline for the flashcard. An example of the command is deadline 3 d/12 January 2020
.
Step 2. Upon adding to a specified flashcard, the deadline 12 January 2020
will be added to the index 3
flashcard.
Step 3. The deadline
command also calls Model#commitFlashBook()
, causing another modified flash book state to be saved into the flashBookStateList
.
Step 4. In the case that a new date is to be added to a flashcard with an existing deadline or note, corrections can be made by overwriting the existing information with the same commands.
Deleting Flashcards
The user now decides that the deadline is over/the note is redundant and do not wish to save the flashcard in the database.
For the user to delete the existing flashcards in the database, there are two options available:
* Option 1: Deleting flashcards by subject. (deleteSubject
command)
* Option 2: Deleting all flashcards. (clear
command)
Option 1: Deleting flashcards by subject. (deleteSubject
command)
By executing the deleteSubject
command, the user can delete all the flashcards under the specified subject. In this case, we will be deleting the subject physics
.
Step 1. When the deleteSubject
command is called, it will be executed by the LogicManager
.
Step 2. Next, it will be parsed through the FlashBookParser
with commandWord deleteSubject()
under parseCommand
.
Step 3. The deleteSubject()
in Model will then be executed and the specified subject physics
will be deleted from the VersionedFlashBook
.
Step 4. The deleteSubject
command calls Model#commitFlashBook()
, causing another modified flash book state to be saved into the flashBookStateList
.
Step 5. The SubjectListPanel
and FlashCardListPanel
will also be updated with new indexes after the deletion.
This action is not reversible. The undo and redo command do not apply to the deletion of subjects.
|
The sequence diagram below illustrate how deleteSubjectCommand
is executed.
image::deleteSubjectSequenceDiagram.png[width="800"]
Option 2: Deleting all flashcards. (clear
command)
By executing the clear
command, all the flashcards will be cleared from the database.
Step 1. When the clear
command is called, it will call Logic#clear()
, which will shift the currentStatePointer
once to the left, pointing it to the previous flash card state, and clear all the respective flash cards in FlashCards.
Step 2. This command also calls Model#commitAddressBook()
.
Step 3. Since the currentStatePointer
is not pointing at the end of the flashBookStateList
, all flash book states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the clear
command. This is the behavior that most modern desktop applications follow.
Step 4. Hence, all flashcards and subjects will be deleted in the database.
Step 5. The FlashBook will then be in a clean slate, at fb3.
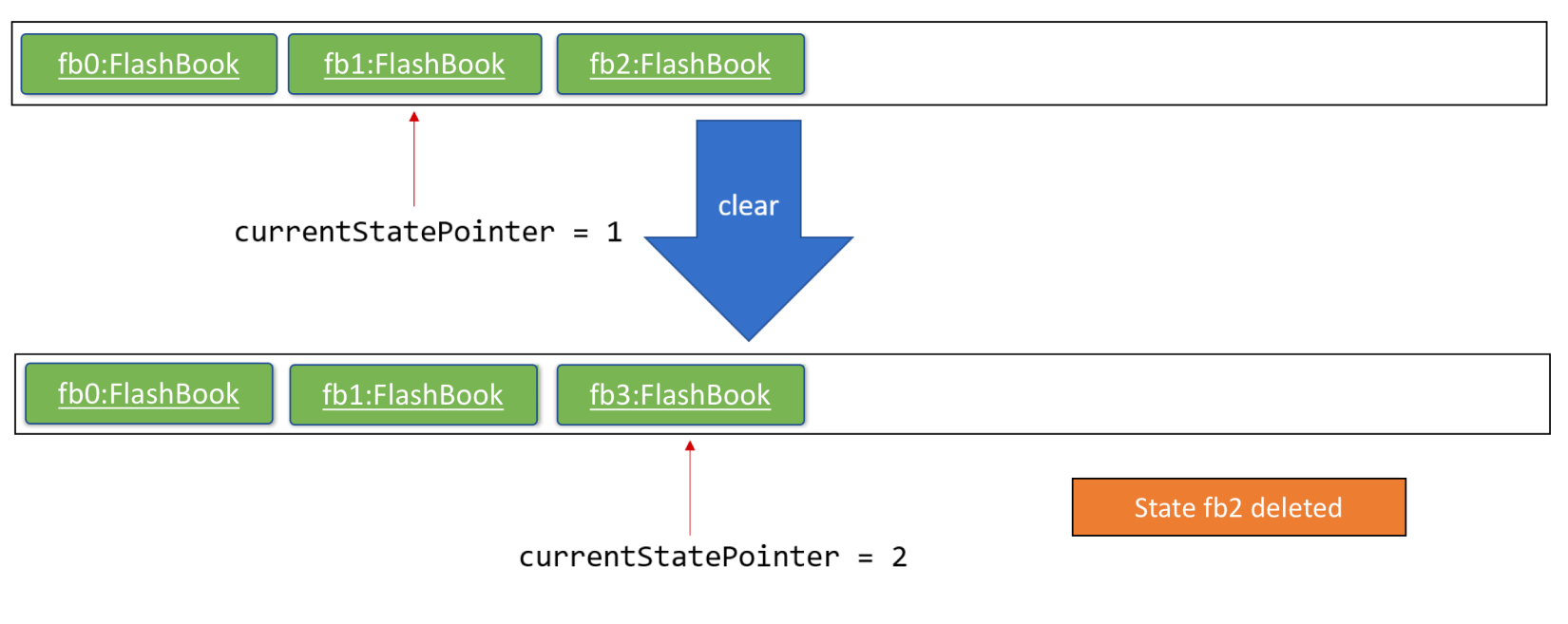
The following activity diagram summarizes what happens when a user executes a 'clear' command:
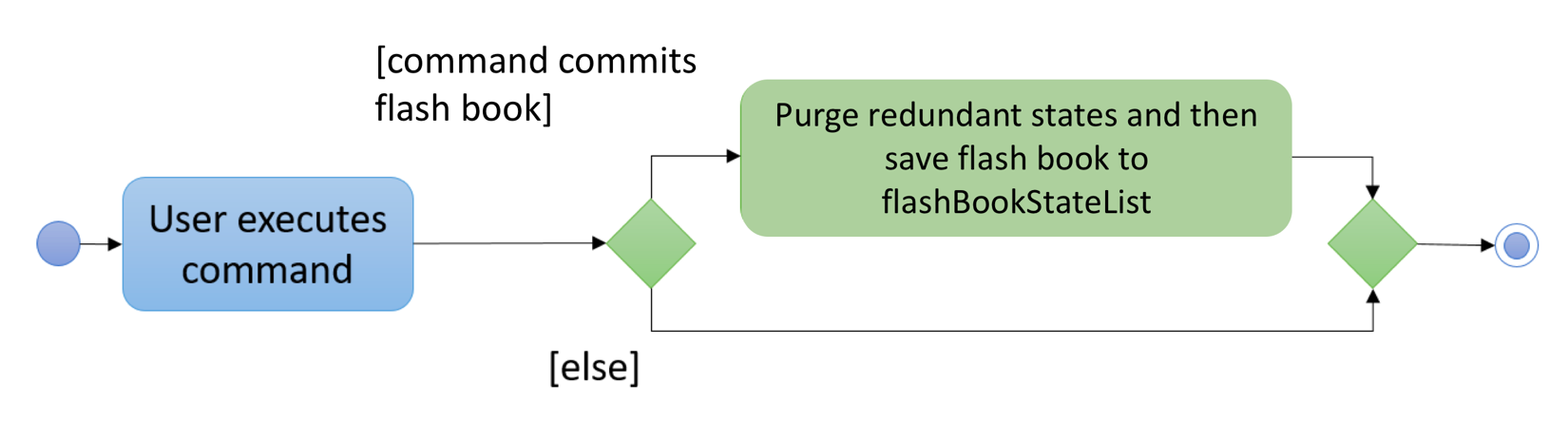
This action is not reversible. The undo and redo command do not apply to the clearing of flashcards and subjects simultaneously.
|
Design Considerations
Aspect: How deadline/note executes
-
Alternative 1 (current choice): Manual deletion of the flashcards.
-
Pros: Allow the user to have the choice of keeping the flashcards or removing it.
-
Cons: Might be troublesome for some users.
-
-
Alternative 2: Auto deletion of the flashcards.
-
Pros: Easy to implement and convenient for the user. Deletes the flashcards right after the deadline.
-
Cons: Need to have additional internal calendar for the auto deletion. E.g. Java Calendar
-
Alternative 1 is chosen to provide users with the choice of keeping and deleting flashcards as and when they want. Even though it will result in overloading of overdue flashcards
and take up excess storage if the user does not manually delete, we designed the command in such a way that it accepts both dates and text. This provides two options for the users:
He/She can choose to write a date or write a note under the flashcard, for example, Clarify with the Teacher
or Edit photosynthesis
. This will allow the user to have the freedom to write whatever they require rather than just deadlines.
Aspect: Data structure to support the deadline/note commands
-
Alternative 1 (current choice): Use the
deleteSubject
orclear
command to manually delete flashcards.-
Pros: Give the user the choice to keep or delete the flashcards. Also, the user can choose to delete the flashcards by subjects or delete all the flashcards altogether.
-
Cons: Both
deleteSubject
andclear
commands cannot be undone once implemented.
-
-
Alternative 2: Implement an internal calendar to calculate the number of days left and auto-deletion of flashcards after the deadline.
-
Pros: Auto deletion of flash cards.
-
Cons: Need to pay extra caution for Leap years. Users do not have the choice of keeping flashcards after the deadline.
-
Alternative 1 is chosen to provide users with the choice of deleting flashcards as and when they want. Even though it will take up extra storage if the user does not manually delete, the user will have better control over what they want to store and delete in the application.